思緒遨遊
將 VC 6 編譯 stl的一堆 warning 消除
加入 #pragma warning(disable:4786) 就可以了
C++ Optimizations 有些是可以注意一下,不過我不認為那是首要要注意的事情
Use Initialization Lists
Always use initialization lists in constructors. For example, use
TMyClass::TMyClass(const TData &data) : m_Data(data)
{
}
rather than
TMyClass::TMyClass(const TData &data)
{
m_Data = data;
}
Without initialization lists, the variable's default constructor is invoked behind-the-scenes prior to the class's constructor, then its assignment operator is invoked. With initialization lists, only the copy constructor is invoked.
Optimize For Loops Wherever possible, count down to zero rather than up to n. For example, use for (i = n-1; i >= 0; --i)
rather than for (i = 0; i < n; ++i)
The test is done every iteration and it's faster to test against zero than anything else. Note also that ++i
is faster than i++
when it appears in the third part of the for loop statement. |
Use 'int' Always use the int data type instead of char or short wherever possible. int is always the native type for the machine. |
Make Local Functions Static Always declare local functions as static, e.g.,
static void foo()
This means they will not be visible to functions outside the .cpp file, and some C++ compilers can take advantage of this in their optimizations. |
Optimize If Statements Factor out jumps. For example, use
bar(); if (condition) { undoBar(); foo(); }
rather than if (condition) { foo(); } else { bar(); }
Use a profiler and good judgement to decide if undoing the bar() operation is faster than jumping. |
Optimize Switch Statements Put the most common cases first. |
Avoid Expensive Operations Addition is cheaper than multiplication and multiplication is cheaper than division. Factor out expensive operations wherever possible. |
Initialize on Declaration Wherever possible, initialize variables at the time they're declared. For example, TMyClass x = data;
is faster than TMyClass x; x = data;
Declaration then initialization invokes the object's default constructor then its assignment operator. Initializing in the declaration invokes only its copy constructor. |
Pass By Reference Always try to pass classes by reference rather than by value. For example, use void foo(TMyClass &x)
rather than void foo(TMyClass x)
|
Delay Variable Declarations Leave variable declarations right until the point when they're needed. Remember that when a variable is declared its constructor is called. This is wasteful if the variable is not used in the current scope. |
Use 'op=' Wherever possible, use 'op=' in favour of 'op'. For example, use x += value;
rather than x = x + value;
The first version is better than the second because it avoids creating a temporary object. |
Inline Small Functions Small, performance critical functions should be inlined using the inline keyword, e.g., inline void foo()
This causes the compiler to duplicate the body of the function in the place it was called from. Inlining large functions can cause cache misses resulting in slower execution times. |
Use Nameless Objects Wherever possible, use nameless objects. For example, foo(TMyClass("abc"));
is faster than TMyClass x("abc"); foo(x);
because, in the first case, the parameter and the object share memory. |
windows
工作列→開始→執行→輸入的指令
gpedit.msc-----群組原則
sndrec32-------錄音機
nslookup-------IP位址偵測器
explorer-------開啟檔案總管
logoff---------登出指令
tsshutdn-------60秒倒計時關機指令
lusrmgr.msc----本地機用戶和組
services.msc---本機服務設定
oobe/msoobe /a----檢查XP是否啟動
notepad--------開啟記事本
cleanmgr-------磁碟垃圾整理
net start messenger----開始信使服務
compmgmt.msc---電腦管理
net stop messenger-----停止信使服務
vconf-----------啟動
dvdplay--------DVD播放器
charmap--------啟動字元對應表
Kdiskmgmt.msc---磁牒管理實用程序
calc-----------啟動電子計算器
dfrg.msc-------磁碟重組工具
chkdsk.exe-----Chkdsk磁牒檢查
devmgmt.msc--- 裝置管理員
bFdrwtsn32------ 系統醫生
srononce -p ----15秒關機
dxdiag---------檢查DirectX資訊
regedt32-------註冊表編輯器
YMsconfig.exe---系統配置實用程序
rsop.msc-------群組原則結果集
mem.exe--------顯示記憶體使用情況
regedit.exe----註冊表
winchat--------XP自帶區域網路聊天
progman--------程序管理器
winmsd---------系統資訊
perfmon.msc----電腦效能監測程序
winver---------檢查Windows版本
sfc /scannow-----掃瞄錯誤並復原
taskmgr-----工作管理器(2000/xp/2003)
eventvwr.msc------------事件檢視器
secpol.msc----------------本機安全性設定
rsop.msc------------------原則的結果集
ntbackup----------------啟動制作備份還原嚮導
mstsc-----------遠端桌面
winver---------檢查Windows版本
wmimgmt.msc----開啟windows管理體系結構(WMI)
wupdmgr--------windows更新程序
wscript--------windows指令碼宿主設定
write----------寫字板
winmsd---------系統資訊
wiaacmgr-------掃瞄儀和照相機嚮導
winchat--------XP原有的區域網路聊天
mem.exe--------顯示記憶體使用情況
sconfig.exe---系統配置實用程序
mplayer2-------簡易widnows media player
mspaint--------畫圖板
mstsc----------遠端桌面連接
mplayer2-------媒體播放機
magnify--------放大鏡實用程序
mmc------------開啟控制台
mobsync--------同步指令
dxdiag---------檢查DirectX資訊
drwtsn32------ 系統醫生
devmgmt.msc--- 裝置管理員
dfrg.msc-------磁碟重組程式
diskmgmt.msc---磁牒管理實用程序
dcomcnfg-------開啟系統元件服務
ddeshare-------開啟DDE共享設定
dvdplay--------DVD播放器
net stop messenger-----停止信使服務
net start messenger----開始信使服務
notepad--------開啟記事本
nslookup-------網路管理的工具嚮導
ntbackup-------系統制作備份和還原
narrator-------螢幕「講述人」
ntmsmgr.msc----移動存儲管理器
ntmsoprq.msc---移動存儲管理員操作請求
netstat -an----(TC)指令檢查連接
Usyncapp--------新增一個公文包
sysedit--------系統配置編輯器
sigverif-------文件簽名驗證程序
psndrec32-------錄音機
shrpubw--------新增共用資料夾
secpol.msc-----本機安全原則
syskey---------系統加密,一旦加密就不能解開,保護windows xp系統的雙重密碼
services.msc---本機服務設定
Sndvol32-------音量控制程序
sfc.exe--------系統檔案檢查器
sfc /scannow---windows文件保護
tsshutdn-------60秒倒計時關機指令
tourstart------xp簡介
taskmgr--------工作管理器
eventvwr-------事件檢視器
eudcedit-------造字程序
explorer-------開啟檔案總管
lpackager-------對像包裝程序
perfmon.msc----電腦效能監測程序
progman--------程序管理器
regedit.exe----註冊表
rsop.msc-------群組原則結果集
rononce -p ----15秒關機
regsvr32 /u *.dll----停止dll文件執行
regsvr32 /u zipfldr.dll------取消ZIP支持
cmd.exe--------CMD命令提示字元
chkdsk.exe-----Chkdsk磁牒檢查
A2V9]certmgr.msc----證書管理實用程序
calc-----------啟動計算器
charmap--------啟動字元對應表
cliconfg-------SQL SERVER 客戶端網路實用程序
Clipbrd--------剪貼板檢視器
conf-----------啟動netmeeting
compmgmt.msc---電腦管理
ciadv.msc------索引服務程序
osk------------開啟螢幕小鍵盤
odbcad32-------ODBC資料來源管理器
oobe/msoobe /a----檢查XP是否啟動
lusrmgr.msc----本地機用戶和組
iexpress-------木馬元件服務工具,系統原有的
fsmgmt.msc-----共用資料夾管理器
utilman--------協助工具管理器
文字處理軟件應該是軟件開發中的一大支柱,而任何軟件中字符串的處理更不可或缺。這裡主要借鑑windows核心編程談談使用UNICODE的好處。
既 然是基於windows編程,就得看看windows平台本身對字符的處理方式。由於ANSI字符採用8位進行編碼,對於西歐ABC之類足夠,然而對於中 東的字符不實用(考慮下我們中國的漢字),所以就出現了UNICODE。window98是基於ANSI的平台,windows2000是基於 UNICODE開發的平台,因此可以知道在調用Windows API的時候,假如我們在98系統上傳遞UNICODE字符,那麼系統在背後會先把字符轉化為ANSI字符然後調用API;相反,我們在2000系統上傳 遞ANSI字符,那麼會先轉化為UNICODE字符。
去年看過(準確說是翻了一下,沒時間看)一本書《C/C++-編程高手箴言》(梁肇 新 超級解霸作者),他裡面有一部分是談到使CPU降溫,很好奇翻了一下,主要講如何使CPU少轉幾圈。我的感想是,要想成為一名優秀的軟件開發人員,必須 make good use of (有時漢語無法表達這麼好) CPU和RAM,儘量少浪費時鐘和內存塊,當然也需要充分利用否則也是浪費,其中把握的是一個度,扯遠了,回來繼續談UNICODE。
先看看使用UNICODE的好處(書上的):
1、可以很容易地在不同語言之間進行數據交換。
2、使你能夠分配支持所有語言的單個二進制。exe文件或DLL文件。
3、提高應用程序的運行效率。
如何基於UNICODE編譯:
只需定義宏_UNICODE或者UNICODE (VC 2005默認採用Unicode編譯)
Windows宏定義處理支持ANSI和UNICODE編譯
大學C、C++語言中我們學習字符表示是char,由於書中講解全部一個模式,而開發類書籍很少講解ANSI、UNICODE字符串區別,使得很少有人關注。在進行函數調用的時候,很少去關注接口處字符串處理,可能無意中你就使CPU多轉幾圈。
ANSI字符表示是char,佔8位;UNICODE字符表示是wchar_t,佔16位。Windows編程用宏對這兩種類型進行了封裝:
typedef char CHAR;
typedef wchar_t WCHAR;表示這兩種字符串數據:
CHAR chANSI[] = "hello";
WCHAR chUnicode[] = L"hello";同樣表示字符串卻要使用兩種表示方法,Windows為我們定義了一套宏用來處理這些問題,例如用TCHAR宏表示字符,用_T()宏來表示字符常量類型,它根據編譯字符集選項來確定具體類型。同樣定義了字符處理函數宏,具體參看msdn。
下面基於提高應用程序的運行效率來探討,純理論分析:
CHAR chANSI[100];
WCHAR chUnicode[100];
// Normal sprintf: all string are ANSI
sprintf(chANSI, "%s", "ANSI Str");
// Converts Unicode string to ANSI (Be careful %s and %S)
sprintf(chANSI, "%S", L"Unicode Str");
// Normal swprintf: all string are Unicode
swprintf(chUnicode, L"%s", L"Unicode Str");
// Converts ANSI string to Unicode (Be careful %s and %S)
swprintf(chUnicode, L"%S", "ANSI Str");從 上面可以看出,簡單的一個打印函數都可能導致CPU多花時鐘來進行函數調用前處理,所以編程時一定要養成好習慣,隨手做到可能使你的代碼與眾不同。由於我 們目前的系統大多是Windows 2000以上版本,採用Uincode字符集,在API調用的時候接口字符都是Unicode的,所以最好採用Unicode字符風格進行編碼,這樣可以 減少調用時轉換開銷。
應該注意的問題:
假設定義一個字符數組TCHAR szName[100],當基於Unicode編譯的時候,它實際佔用200字節,假設有一個給字符賦值函數:
void SetName(TCHAR* pName, int iSize)調用該函數:
SetName(szName, sizeof(szName))這樣就可能產生錯誤,sizeof求出的是數組所佔字節數目,而不是字符個數,字符個數應該是sizeof(szName)/sizeof(TCHAR)。所以編程的時候腦袋一定要繃緊一根弦,提防類似錯誤。
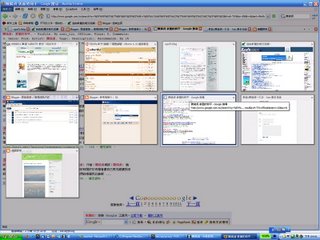
好用的 Tab Catalog
按一個鍵就讓你的電腦擁有像是mac的錯覺
頗適合大螢幕的電腦
去這網站下載firefox真是好物
有很多有趣實用的extension可用
而且光是不會像IE被綁架 就省去我許多的麻煩
好幾個同事已經在我的影響下 轉投firefox的陣營了
<太多>
喜歡一個人孤獨的時刻
但不能喜歡太多
在地鐵站或美術館
孤獨像睡眠一樣餵養我
以永無止盡的墜落
需要音樂取暖
喜歡一個人孤獨的時刻
但不能喜歡太多
喜歡一個喝著紅酒的女孩
在下雨音樂奏起的時候
把她送上鐵塔給全世界的人寫明信片
像一隻鳥在最高的地方
歌聲嘹喨
喜歡一個喝著紅酒的女孩
但不能喜歡太多
喜歡一個陽光照射的角落
但不能喜歡太多
是幼稚園的小朋友
笑聲像睡眠一樣打擾我
我們輕輕的揮一揮手
凝結照片的傷口
我喜歡一個陽光照射的角落
但不能喜歡太多
喜歡一個人孤獨的時刻
但不能喜歡
太多
旅行的意義 你看過了許多美景 你看過了許多美女 你迷失在地圖上 每一道短暫的光陰 你品嚐了夜的巴黎 你踏過下雪的北京 你熟記書本裡 每一句你最愛的真理 卻說不出你愛我的原因 卻說不出你欣賞我哪一種表情 卻說不出在什麼場合我曾讓你動心 說不出離開的原因 你累計了許多飛行 你用心挑選紀念品 你蒐集了地圖上 每一次的風和日麗 你擁抱熱情的島嶼 你埋葬記憶的土耳其 你流連電影里美麗的不真實的場景 卻說不出你愛我的原因 卻說不出你欣賞我哪一種表情 卻說不出在什麼場合我曾讓你分心 說不出旅行的意義 勉強說出你為我寄出的每一封信 都是你離開的原因 你離開我 就是旅行的意義
又聽到朋友分手的消息了 秋天真是分手的季節.. 在一起六年的感情阿
看來是因為工作太認真而忽略了她 他說他們約會去咖啡廳時 他常常拿出書來研讀 追求自己的成長卓越 最後還跟我說當初她是如何愛他阿
不過 女生一句話 "感覺變了" 就打死一個人囉
所以在科技業的男士們 別讓自己成為好人了
多認識一些女孩吧